다중참조와 매개변수
이를테면 평소의 일상적인 상식과 별반 다를 것이 없는 이유로 대충 넘어감.
p.219_1
#include <stdio.h>
int main(void)
{
float *fp, **mfp, val;
fp = &val; //val pointing
mfp = &fp; //fp pointing
**mfp = 123.903; //역참조
printf("%f %f \n", val, **mfp);
printf("fp %p %p\n", &fp, fp);
printf("mfp %p %p\n", &mfp, mfp);
printf("val %p\n", &val);
return 0;
}
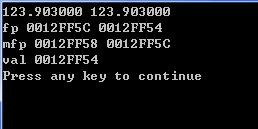
p.219_2
#include <stdio.h>
int main(void)
{
char *p, **mp, str[80];
p = str; //str[80] pointing
mp = &p; //p pointing
printf("Enter your name: ");
gets(*mp); //mp enter string
printf("Hi %s. ", *mp); //mp output
return 0;
}
p.221_1
#include <stdio.h>
void myputs(char *p);
int main(void)
{
myputs("this is a test");
return 0;
}
void myputs(char *p)
{
while(*p) {
printf("%c", *p++);
//p++; //p가 가리키는 주소를 증가
}
printf("\n");
}
p.221_2
#include <stdio.h>
void myputs(char *to, char *from);
int main(void)
{
char str[80];
myputs(str, "this is test");
printf(str);
return 0;
}
void myputs(char *to, char *from)
{
while(*from) *to++ = *from++;
*to = '\0';
}